"Art is never finished, only abandoned"
A few things I've learned along the way
I have been working on a very simple, low-cost functional, compact EV charger; here's my ramblings on what I've got so far.
The Plug
I can't make my own plug easily. I'm certain I can get one built, and it would work, but the risk and quality controls required to make the complex receptacle are too beyond my scope.
The Circuit Analysis
My first version of the square wave level-shifter (5v -> 12v) just used a very simple inverted NPN configuration. It is very simple, but wastes a fair amount of current when the circuit is dropped. Also, when gate closes the circuit the output voltage was about 27mv. There's always going to be some leak voltage in this configuration.
 |
! NOTE - ONLY SEMI - WORKS ! |
So, I own a Nissan Leaf and a Chevy Volt. I plugged everything up and the Volt worked great, the Leaf... Never got beyond the "plugged-in" beep. So it never recognized my +26mv to +12v square wave as the pilot function. Oddly a very early test used a simple Op-Amp for this level-shift and the Leaf did recognize that form.
Cuiriouser and curiouser... After analyzing in CircuitLabs, I found the op-amp version did not leak and actually went -2mv. I do not have the equipement to test this experimentally, so I am unsure if this is a simulation effect or an actual LRC overshoot. Either way, the Leaf did accept this signal as accurate
Additonal research: I think a "high side switch" (HSS) NPN/PNP configuration could also work better. It was more efficient as it wasn't trying to sink 12v to gound through a 1k. It got as low as 2mv, much closer, but not drop through zero as the op-amp did. I knew the op-amp worked before, simulated better, so my v2 uses the op-amp.
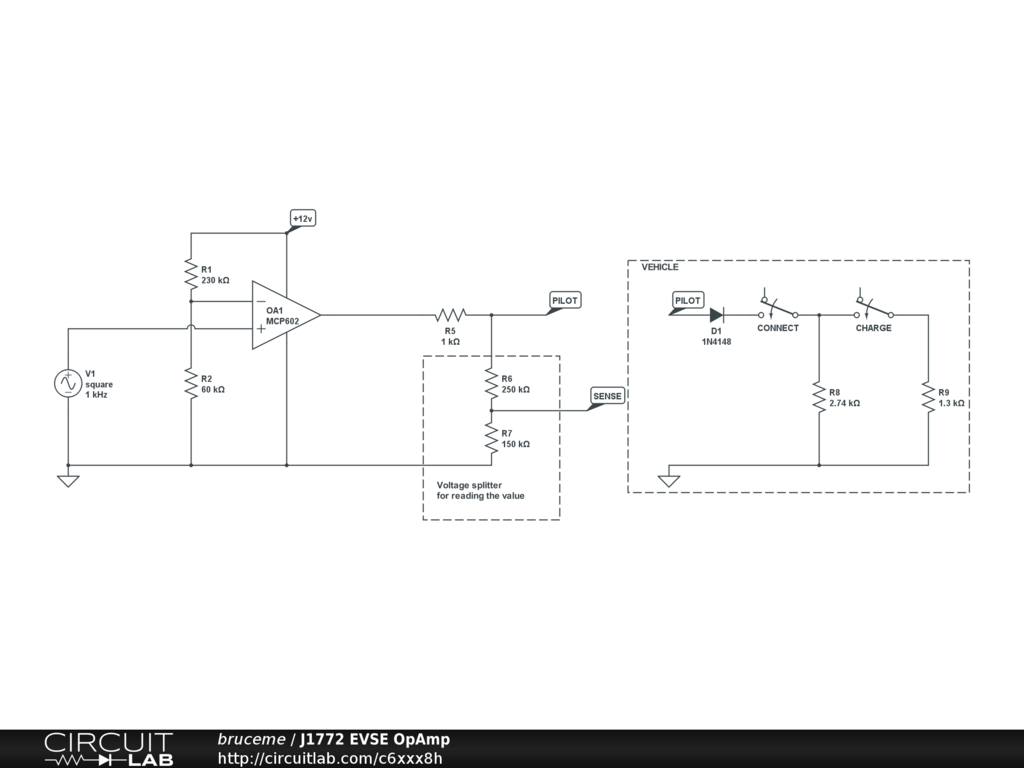 |
This version works! |
Link to CircuitLab TinyEVSE circuit
Micro Controller
I chose the ATTiny85 for all these reasons:
- Very inexpensive (~$.80 in quantity)
- It has all the power of much larger MC
- Shares much of the same traits of the wildly popular Arduino processors ATMega328
- Small 8-pin package
- Very low power
- Very simple to configure, with an internal clock that's accurate enough
Because TinyEVSE has very low processing requirements, I lowered the internal clock to 1mhz, to save power.
Code
It's really not complicated... Here's an overview;
you can find it here
It all starts with a 1000hz (1ms) square wave, the ATTiny85's built in hardware PWM is the best choice for generating this wave. Some research and you can download some apps to tell you how to configure that. To use OCR1B on PB4 1khz frequency, you need to set;
// 1khz Timer1 (PB4)
OCR1B = 250;
TCCR1 = 0x83;
The duty cycle of the wave sets EVSE's request current. analogWrite() achieves this.
// set EVSE current capacity in Amperes
// see: https://code.google.com/p/open-evse/wiki/J1772Basics
//
void SetCurrent(int amps)
{
analogWrite(PILOT_PIN_OUT, 256 * BoundAmps(amps) / 60);
}
If you do the math, duty cycle is requested amps / 0.6 in %. So if I am requestig 15A; 25% duty.
This square wave signal is run through a level-shift to 12v to the car. The car then chops the +12v to +9v. That tells me, we've plugged in. Then the car further chops the signal to +6v. That's my signal to get in gear and energize the relay.
I do the sense line / EVSE state analysis using a short 100 iteration min/max polling loop on the sense in GetPilotMax(). That output is fed into GetState(int pilot) which "de-bounce" filter the output the true current EVSE state. This removes the chance that random signals cause eronious state changes.
At the highest level this code snip sums it up nicely:
if (HasStateChanged(GetState(GetPilotMax())))
{
SetCurrent(currentRate);
if (EVSE_PILOT_STATE_C == evsePilotState)
StartCharging();
else
StopCharging();
}
Program the board
I built a programmer to flash my controller directly. I used a UsbTinyISP, you can get them on eBay cheaply. You can program this using the Arduino IDE, but I have fallen in love with CodeBender.cc
Board
I have spent WAY WAY WAY too much time tinkering on EagleSoft CAD. I have made at least a dozen versions of this. Some use 12v DC Relays, some used 240vac 2P contactors. Ultimately I went with the cheaper compact 12v relays because I can run them on 120/240VAC. They are marginally simpler and marginally cheaper. But I do think a 2P 240vac contactor could work really well using an optical SCR to energize the coil and it would last much longer. But it is much larger than the PB 12v relays.
A few criteria for the board design;
- Simple
- Compact
- Convenient to assemble; connects directly to the relay pins
- Single sided so I could etch it myself
- Huge over sized traces anyone (even me) could etch without issue
 |
Final Artwork |
Eagle Files:
Schematic
Board
So build it already!
To make a "TinyEVSE" the best thing to do;
- Buy a plug on eBay, they sell for about $100.
- Build a TinyEVSE controller board for a ATTiny85; $20
- Buy a relay $7
- Buy/Convert a small 12v power supply (200ma+)
- Buy or build a box
So total cost to build your own 30A Level 2 EVSE; well under $150.
Formal BOM :
DIP
Part | Value | Device | Package | Description | PROD_ID | VALUE |
U1 | TINY85-20-DIP | ATTINY45TINY85-20-DIP | DIP08 | Atmel 8-pin 2/4/8kB flash uC | IC-09445 | TINY85-20-
|
IC1 | LM340MP-05 | LM340MP-05 | SOT223 | Positive VOLTAGE REGULATOR | | |
IC2 | LM321MF | LM321MF | SOT23-5 | Low Power Single Op Amp | | |
Q1 | MMBT5088 | TRANSISTOR_NPNSOT23-I | SOT23 | Generic NPN BJT | | |
D1 | | DIODESMB | SMB-DIODE | Diode | DIO-09646 | |
C2 | 1.0uF | 1.0UF-25V-+80/-20(0805) | 0805 | CAP-11625 | CAP-11625 | 1.0uF |
R1 | 20k | 0.75OHM1/4W1%(0805) | 0805 | RES-08474 | RES-08474 | 0.75 |
R2 | 1k | 0.75OHM1/4W1%(0805) | 0805 | RES-08474 | RES-08474 | 0.75 |
R3 | 10k | 0.75OHM1/4W1%(0805) | 0805 | RES-08474 | RES-08474 | 0.75 |
R4 | 200k | R-US_MELF0204R | MINI_MELF-0204R | RESISTOR, American symbol | | |
R5 | 120k | 0.75OHM1/4W1%(0805) | 0805 | RES-08474 | RES-08474 | 0.75 |
R6 | 1k | 0.75OHM1/4W1%(0805) | 0805 | RES-08474 | RES-08474 | 0.75 |